- Docs For Xcode Free
- Xcode Help
- Docs For Xcode Editor
- Apple Id Developer Account
- Developer Xcode Download
- Generate xcode project for debugging sources (cannot build code from xcode) Run gn gen with the -ide=xcode argument. $ gn gen out/Testing -ide=xcode This will generate the electron.ninja.xcworkspace. You will have to open this workspace to set breakpoints and inspect. See gn help gen for more information on generating IDE projects with GN.
- Xcode’s Quick Help, Documentation and API Docs. Xcode includes documentation, and documentation tools, right within the IDE. We’ve already seen the Quick Help Inspector in this tutorial, but there’s more! Documentation about your code, such as quick help annotations and code comments.
The ability to quickly access documentation is a major feature of Xcode, and one you’ll want to use regularly for your iOS app project. If you have no idea how to do something, or how something works, you can often find the answer in the documentation. Being able to figure out what’s going on will. Now, navigate to your Xcode project folder and you will see a folder ‘docs’.Open index.html from this folder and you will get your Apple like document site. The LANG environment variable is not set in any location that your Xcode can reach. Probably because it wasn't set in your build settings. Running the command below will print all environment variables, LANG will not be among them. Xcodebuild -project Path/To/Your.xcodeproj -target 'YourTarget' -showBuildSettings.
Code structure and organization is a matter of pride for developers. Clear and consistent code signifies clear and consistent thought. Even though the compiler lacks a discerning palate when it comes to naming, whitespace, or documentation, it makes all the difference for human collaborators.
This week, we’ll be documenting the here and now of documentation in Swift.
Since the early ’00s, Headerdoc has been Apple’s preferred documentation standard. Starting off as little more than a Perl script that parsed trumped-up Javadoc comments, Headerdoc would eventually be the engine behind Apple’s developer documentation online and in Xcode.
But like so much of the Apple developer ecosystem, Swift changed everything. In the spirit of “Out with the old, in with the new”, Xcode 7 traded Headerdoc for fan favorite Markdown — specifically, Swift-flavored Markdown.
Documentation Comments & Swift-Flavored Markdown
Even if you’ve never written a line of Markdown before, you can get up to speed in just a few minutes. Here’s pretty much everything you need to know:
Basic Markup
Documentation comments look like normal comments, but with a little something extra. Single-line documentation comments have three slashes (///
). Multi-line documentation comments have an extra star in their opening delimiter (/** ... */
).
Standard Markdown rules apply inside documentation comments:
- Paragraphs are separated by blank lines.
- Unordered lists are marked by bullet characters (
-
,+
,*
, or•
). - Ordered lists use numerals (1, 2, 3, …) followed by either a period (
1.
) or a right parenthesis (1)
). - Headers are preceded by
#
signs or underlined with=
or-
. - Both links and images work, with web-based images pulled down and displayed directly in Xcode.
Summary & Description
The leading paragraph of a documentation comment becomes the documentation Summary. Any additional content is grouped together into the Discussion section.
If a documentation comment starts with anything other than a paragraph, all of its content is put into the Discussion.
Parameters & Return Values
Xcode recognizes a few special fields and makes them separate from a symbol’s description. The parameters, return value, and throws sections are broken out in the Quick Help popover and inspector when styled as a bulleted item followed by a colon (:
).
- Parameters: Start the line with
Parameter <param name>:
and the description of the parameter. - Return values: Start the line with
Returns:
and information about the return value. - Thrown errors: Start the line with
Throws:
and a description of the errors that can be thrown. Since Swift doesn’t type-check thrown errors beyondError
conformance, it’s especially important to document errors properly.
Docs For Xcode Free
Are you documenting a function whose method signature has more arguments than a Hacker News thread about tabs vs. spaces? Break out your parameters into a bulleted list underneath a Parameters:
callout:
Additional Fields
In addition to Parameters
, Throws
and Returns
, Swift-flavored Markdown defines a handful of other fields, which can be loosely organized in the following way:
- Algorithm/Safety Information
Precondition
Postcondition
Requires
Invariant
Complexity
Important
Warning
- Metadata
Author
Authors
Copyright
Date
SeeAlso
Since
Version
- General Notes & Exhortations
Attention
Bug
Experiment
Note
Remark
ToDo
Each of these fields is rendered in Quick Help as a bold header followed by a block of text:
Field Header:
The text of the subfield is displayed starting on the next line.
Code blocks
Demonstrate the proper usage or implementation details of a function by embedding code blocks. Inset code blocks by at least four spaces:
Fenced code blocks are also recognized, delimited by either three backticks (`
) or tildes (~
):
Documentation Is My New Bicycle
How does this look when applied to an entire class? Quite nice, actually!
Option-click on the initializer declaration, and the description renders beautifully with a bulleted list:

Open Quick Documentation for the method travel
, and the parameter is parsed out into a separate field, as expected:
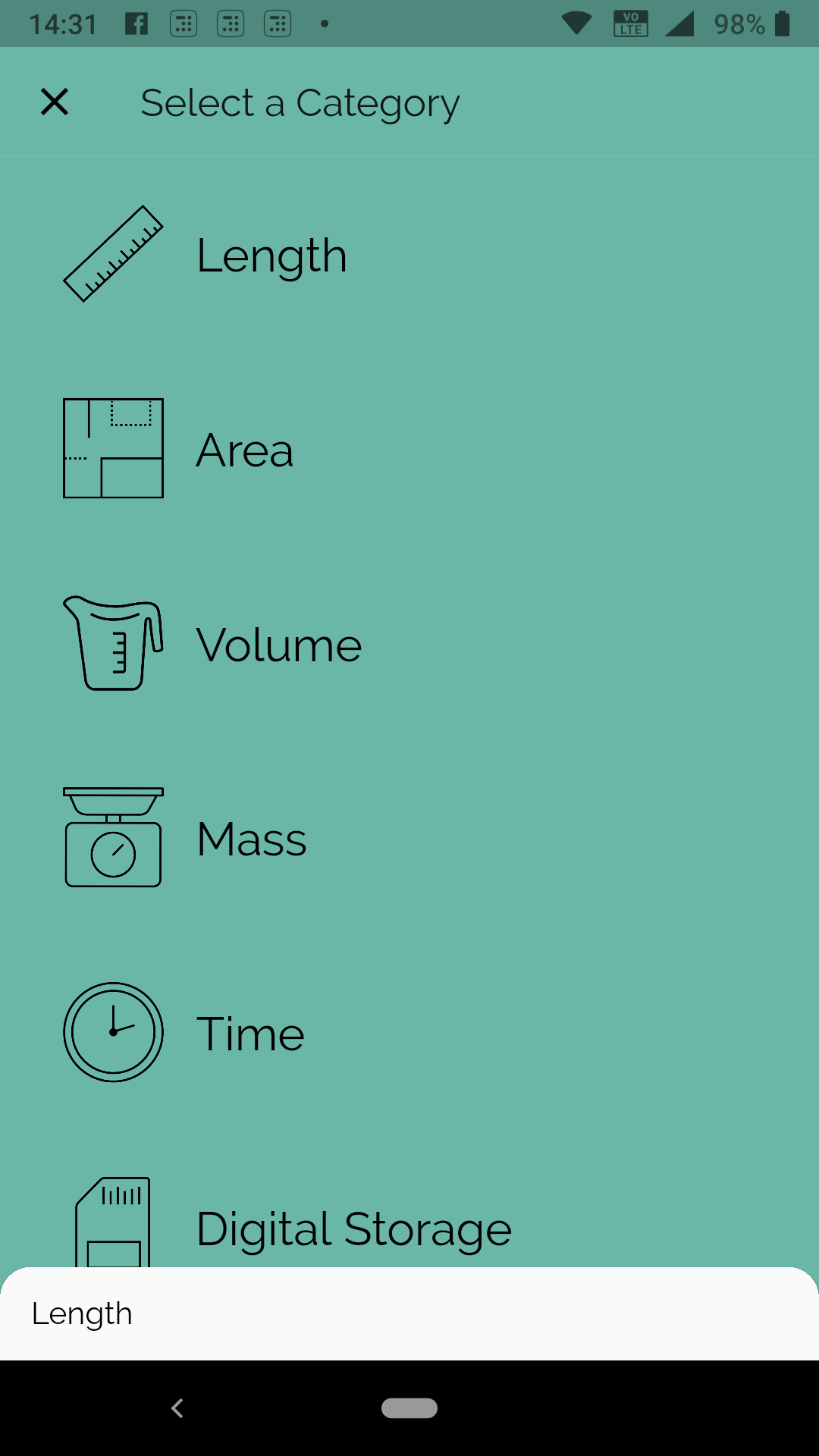
MARK / TODO / FIXME
In Objective-C, the preprocessor directive #pragma mark
is used to divide functionality into meaningful, easy-to-navigate sections. In Swift, the same can be accomplished with the comment // MARK:
.
The following comments are surfaced in the Xcode source navigator:
// MARK:
// TODO:
// FIXME:
Other conventional comment tags, such as NOTE
and XXX
aren’t recognized by Xcode.
As with #pragma
, marks followed by a single dash (-
) are preceded with a horizontal divider
To show these new tags in action, here’s how the Bicycle
class could be extended to adopt the CustomStringConvertible
protocol, and implement the description
property.
Bringing everything together in code:
At the time of writing, there’s no official tool for transforming documentation comments into something more tangible than Quick Help panels in Xcode,
Fortunately, where necessity arises, open source (often) delivers.
Jazzy
Jazzy is a terrific open-source command-line utility that transforms your project’s documentation comments into a set of Apple-like HTML documentation (but that nice vintage style, before that whole redesign). Jazzy uses Xcode’s SourceKitService to read your beautifully written type and method descriptions.
Install Jazzy as a gem, then run from the root of your project folder to generate documentation.

Take a peek at a Jazzy-generated documentation for the Bicycle
class.
Although the tooling and documentation around Swift is still developing, one would be wise to adopt good habits early, by using the new Markdown capabilities for documentation, as well as MARK:
comments in Swift code going forward.
Go ahead and add it to your TODO:
list.
Azure Pipelines | Azure DevOps Server 2020 | Azure DevOps Server 2019 | TFS 2018 | TFS 2017
This guidance explains how to automatically build Xcode projects.
Example
For a working example of how to build an app with Xcode, import (into Azure Repos or TFS) or fork (into GitHub) this repo:
The sample code includes an azure-pipelines.yml
file at the root of the repository. You can use this file to build the app.
Follow all the instructions in Create your first pipeline to create a build pipeline for the sample app.
Build environment
You can use Azure Pipelines to build your apps with Xcode without needing to set up any infrastructure of your own. Xcode is preinstalled on Microsoft-hosted macOS agents in Azure Pipelines. You can use the macOS agents to run your builds.
For the exact versions of Xcode that are preinstalled, refer to Microsoft-hosted agents.
Create a file named azure-pipelines.yml in the root of your repository. Then, add the following snippet to your azure-pipelines.yml
file to select the appropriate agent pool:
Build an app with Xcode
To build an app with Xcode, add the following snippet to your azure-pipelines.yml
file. This is a minimal snippet for building an iOS project using its default scheme, for the Simulator, and without packaging. Change values to match your project configuration. See the Xcode task for more about these options.
Signing and provisioning
An Xcode app must be signed and provisioned to run on a device or be published to the App Store. The signing and provisioning process needs access to your P12 signing certificate and one or more provisioning profiles. The Install Apple Certificate and Install Apple Provisioning Profile tasks make these available to Xcode during a build.
The following snippet installs an Apple P12 certificate and provisioning profile in the build agent's Keychain. Then, it builds, signs, and provisions the app with Xcode. Finally, the certificate and provisioning profile are automatically removed from the Keychain at the end of the build, regardless of whether the build succeeded or failed. For more details, see Sign your mobile app during CI.
CocoaPods
If your project uses CocoaPods, you can run CocoaPods commands in your pipeline using a script, or with the CocoaPods task. The task optionally runs pod repo update
, then runs pod install
, and allows you to set a custom project directory. Following are common examples of using both.
Carthage
If your project uses Carthage with a private Carthage repository,you can set up authentication by setting an environment variable namedGITHUB_ACCESS_TOKEN
with a value of a token that has access to the repository.Carthage will automatically detect and use this environment variable.
Xcode Help
Do not add the secret token directly to your pipeline YAML.Instead, create a new pipeline variable with its lock enabled on the Variables pane to encrypt this value.See secret variables.
Here is an example that uses a secret variable named myGitHubAccessToken
for the value of the GITHUB_ACCESS_TOKEN
environment variable.
Testing on Azure-hosted devices
Add the App Center Test task to test the app in a hosted lab of iOS and Android devices. An App Center free trial is required which must later be converted to paid.
Sign up with App Center first.
Retain artifacts with the build record
Add the Copy Files and Publish Build Artifacts tasksto store your IPA with the build record or test and deploy it in subsequent pipelines. See Artifacts.
Deploy
App Center
Add the App Center Distribute task to distribute an app to a group of testers or beta users,or promote the app to Intune or the Apple App Store. A free App Center account is required (no payment is necessary).
Docs For Xcode Editor
Apple App Store
Install the Apple App Store extensionand use the following tasks to automate interaction with the App Store. By default, these tasks authenticate to Appleusing a service connection that you configure.
Release
Add the App Store Releasetask to automate the release of updates to existing iOS TestFlight beta apps or production apps in the App Store.
See limitationsof using this task with Apple two-factor authentication,since Apple authentication is region specific andfastlane session tokens expire quickly and must be recreated and reconfigured.
Promote
Add the App Store Promotetask to automate the promotion of a previously submitted app from iTunes Connect to the App Store.
Apple Id Developer Account
Related extensions
Developer Xcode Download
- Apple App Store (Microsoft)
- Codified Security (Codified Security)
- MacinCloud (Moboware Inc.)
- Mobile App Tasks for iOS and Android (James Montemagno)
- Mobile Testing Lab (Perfecto Mobile)
- Raygun (Raygun)
- React Native (Microsoft)
- Version Setter (Tom Gilder)
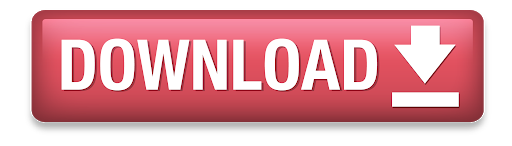